[toc]
图像处理
旋转图片
1 | import cv2 |
python函数模块化,并安装在本地调用
- 首先,把编写好的函数写在一个指定文件(example:xxx.py)里,并放到一个文件夹(example:xxx)里。
- 接着,在这个文件夹里写一个setup.py文件,文件内容例如:
1 | $ from distutils.core import setup |
- 打包前MANIFEST.in包括和排除文件/文件夹
1 | # 包含匹配指定模式的文件 |
- 然后,在此文件夹里打开cmd,输入:
1 | $ python setup.py sdist |
- 最后,安装此模块到本地,输入:
1 | $ pip install . |
python包上传至PYPI
安装
setuptools
和wheel
库,这两个库是 Python 的包打包工具,可以用来生成安装包和源码包,twine
用来上传PyPI:1
2pip install setuptools wheel
pip install twine编写
setup.py
文件,这个文件描述了项目的元数据和打包方式。这个文件通常位于项目的根目录下,下面是一个示例:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20"""run this
python setup.py sdist
pip install .
"""
# from distutils.core import setup
from setuptools import setup, find_packages
setup(
name = 'ReadLog',
version = '1.1.0',
py_modules = ['ReadLog'],
author = 'CHENDONGSHENG',
author_email = 'eastsheng@hotmail.com',
packages=find_packages('src'),
package_dir={'': 'src'},
install_requires=open('requirements.txt').readlines(),
url = 'https://github.com/eastsheng/ReadLog',
description = 'Read themo info from lammps output file or log file'
)在项目的根目录下执行以下命令生成源码包和安装包:
1
2python setup.py sdist
twine upload dist/*
打包Python程序为exe
打包后可以在Windows上运行,而不需要安装程序里需要的各种包。
(1). 安装第三方包pyinstaller
(2). 在cmd命令行输入:
1 | pip install pyinstaller |
(3). 如果嫌慢,或者失败,可以用国内镜像网址下载安装:
1 | pip install pyinstaller -i https://pypi.douban.com/simple |
或者设置Windows国内镜像,具体参考这里:
在 windows “文件资源管理器” 地址栏 输入
%APPDATA%
回车;或者直接找到路径C:\Users\yourcomputer\AppData\Roaming
创建名为
pip
的文件夹在里面新建名为
pip.ini
的配置文件在 pip.ini 文件中输入以下内容,然后保存
1
2
3
4[global]
index-url=http://mirrors.aliyun.com/pypi/simple/
[install]
trusted-host=mirrors.aliyun.com然后可以试试快不快
(4). cmd执行:
1 | pyinstaller -F filename.py |
(5). 还可以给程序加个图标:
1 | pyinstaller -i <图标名.ico> -F filename.py |
(6). 其他需要用到的命令
1 | pyinstaller -h 为查看help命令 |
turtle画太极
画太极;打包后的exe文件
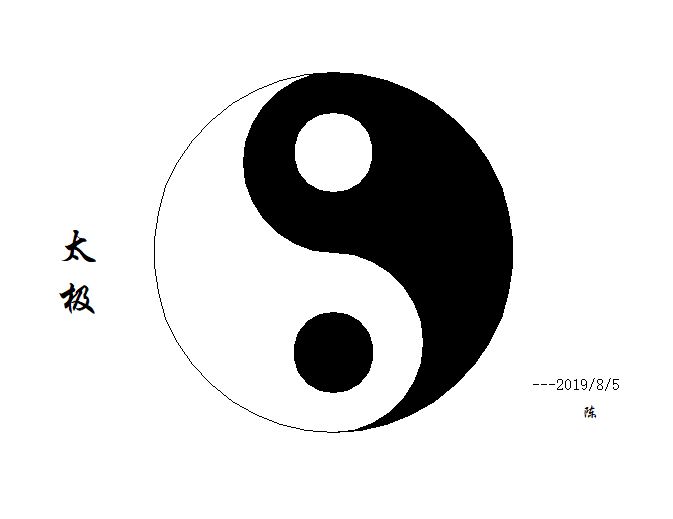
1 | #画个太极阴阳鱼 |
if name == ‘main‘:
1 | if __name__ == '__main__': |
- 为什么有的执行代码前都要写一个if
__name__
是Python
中一个隐含的变量它代表了模块的名字- 只有被
Python
解释器直接执行的模块的名字才是__main__
- 也即是,除非直接执行该代码,否则是不会执行
if __name__ == '__main__':
下面的代码的。 if __name__ == '__main__':
下的变量是全局变量
文件、路径后缀等操作
os.path.split() 分离文件路径与文件名
1 | import os |
os.path.splitext() 分离文件名与后缀
1 | import os |
字典操作
定义字典
1 | HeavyOil_dict ={ |
字典键索引值
1 | HeavyOil_dict["Sat"] |
字典不能索引
- 字典不能索引,可以直接改为
list
后索引1
HeavyOil_Label = list(HeavyOil_dict.keys())
List操作
去除list的括号与引号
1 |
|
列表解析式
1 | #普通 |
count某个元素在列表中出现的次数
1 | some_list = ['a', 'b', 'c', 'b', 'd', 'm', 'n', 'n'] |
Useful Python Librarys
- 用于制作简单 GUI 程序的 Python 库。换一种简单的方式写 GUI(图形用户界面)程序
1 | from guietta import _, Gui, Quit |
导出与安装requirements.txt
导出环境中所有依赖库
1
pip freeze > requirements.txt
指定项目文件夹中依赖库
1
2pip install pipreqs
pipreqs xxx ## xxx代表项目路径在其他环境安装
requirements.txt
中依赖库1
pip install -r requirements.txt
运行Python时传入参数
具体实现
- 如下
run.py
:1
2
3
4
5import sys
x = sys.argv[1] # 传入参数1
y = sys.argv[2] # 传入参数2
print("第一个参数为:",x)
print("第二个参数为:",y) - 具体运行方法:
1
python run.py 1 2
- output
1
2第一个参数为: 1
第二个参数为: 2
若要可以直接运行脚本
- 如在Linux中运行,可在脚本中添加
#!/usr/bin/python
1
2
3
4
5
6#!/usr/bin/python
import sys
x = sys.argv[1] # 传入参数1
y = sys.argv[2] # 传入参数2
print("第一个参数为:",x)
print("第二个参数为:",y) - 若要可执行,还需给脚本设置权限
1
chmod +x ./run.py # 视情况添加sudo
Python调用其他语言
调用C/C++
1. CTypes
- 参考:https://zhuanlan.zhihu.com/p/71243212
- 参考:https://physicspython.wordpress.com/2020/11/11/using-c-functions-in-python/
- 生成
test.so
动态链接库文件:1
g++ -o test.so -shared -fPIC test.cpp
- Python调用C++
1 | import ctypes |
2. SWIG
3. Python/C API
4. Cython
调用Fortran
使用numpy中的f2py
hello_fortran.f90
1 | subroutine hello (a) |
使用
f2py
编译fortran1
f2py -m hello_fortran -c hello_fortran.f90
python中调用
1
2import hello_fortran as hf
hf.hello(45)
减少内存占用
__slots__例子:
- 创建许多对象(成千上万个),它会消耗掉很多内存
1
2
3
4
5
6
7class MyClass(object):
__slots__ = ['name', 'identifier']
def __init__(self, name, identifier):
self.name = name
self.identifier = identifier
self.set_up()
# ...
pypy安装
Linux命令行安装pypy和pypy3
1 | sudo apt-get install pypy pypy-dev #pypy2 |
Linux手动安装pypy3
- 官网下载最新的pypy版本,直接解压
tar -xf pypy3.8-v7.3.7-linux64.tar.bz2
- 创建软链:
sudo ln -s /root/pypy3-v6.0.0-linux64/bin/pypy3 /usr/local/bin
创建软链 - pypy安装成功,输入pypy3测试 pypy3 xxx.py 运行python文件
- 然后开始安装pip命令
- 下载
wget https://bootstrap.pypa.io/get-pip.py
- 然后执行
pypy3 get-pip.py
- 测试pip是否安装成功
pypy -m pip install numpy
- 下载
- 还可安装自己的库:
1
pypy3 setup.py install
卸载pypy
- 完全卸载pypy(软件及相关配置)
1
sudo apt-get remove --purge pypy
- 完全卸载pypy及其依赖软件(慎用!这里会删除pypy及依赖pypy的软件包,一般上面第一条命令已经够用)
1
2sudo apt-get remove --auto-remove pypy
sudo apt-get purge --auto-remove pypy - 清除pypy及其依赖软件的安装包
1
sudo apt-get autoclean pypy
BUG
- 部分第三方库不兼容
- 从此处查看
参考
自动给数字前面补0的方法
1 | n = "123" |
一款 AI 修复神器Lama Cleaner
- 安装好后,就可以启动一个 web 服务了。
1
2
3
4pip install lama-cleaner
# Models will be downloaded at first time used
lama-cleaner --model=lama --device=cpu --port=8080
# Lama Cleaner is now running at http://localhost:8080
查找路径下目录或文件glob
1 | """ |